Logout issues in using multiple tabs in ReactJS or NextJs: BroadcastChannel
BroadcastChannel is a JavaScript API that allows communication between different windows, tabs, or frames that are under the same origin. It provides a simple way to broadcast messages to multiple recipients and facilitates inter-tab communication in web applications.
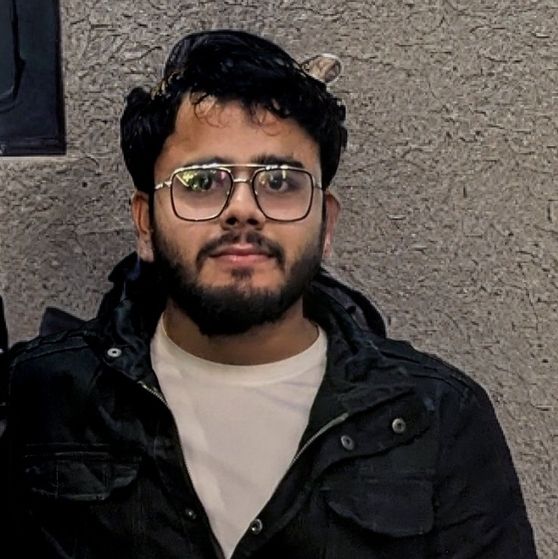
Blog post by Mohit kandhari - Published at 8/5/2023, 6:45:49 PM
The main use case for BroadcastChannel is to enable real-time communication and synchronization of data between different instances of the same web application running in different tabs or windows of a browser. This can be especially useful for scenarios like multi-tab applications, collaborative editing, or keeping different tabs in sync.
Here's a basic overview of how BroadcastChannel works:
Logout issues in applications with multiple tabs can occur when a user logs out in one tab, but the other tabs still remain logged in. This can lead to inconsistent behavior and security vulnerabilities. The Broadcast Channel API can be used to communicate between different tabs and synchronize actions like logging out. Here's how you can use the Broadcast Channel API to resolve logout issues in a ReactJS or NextJS application:
Implement Logout Broadcast: In your application, when a user initiates a logout action, you should broadcast a message to all other tabs using the Broadcast Channel API. Here's an example of how you might do this:
create a custom hook called useBroadcastChannel in a React application to simplify using the Broadcast Channel API:
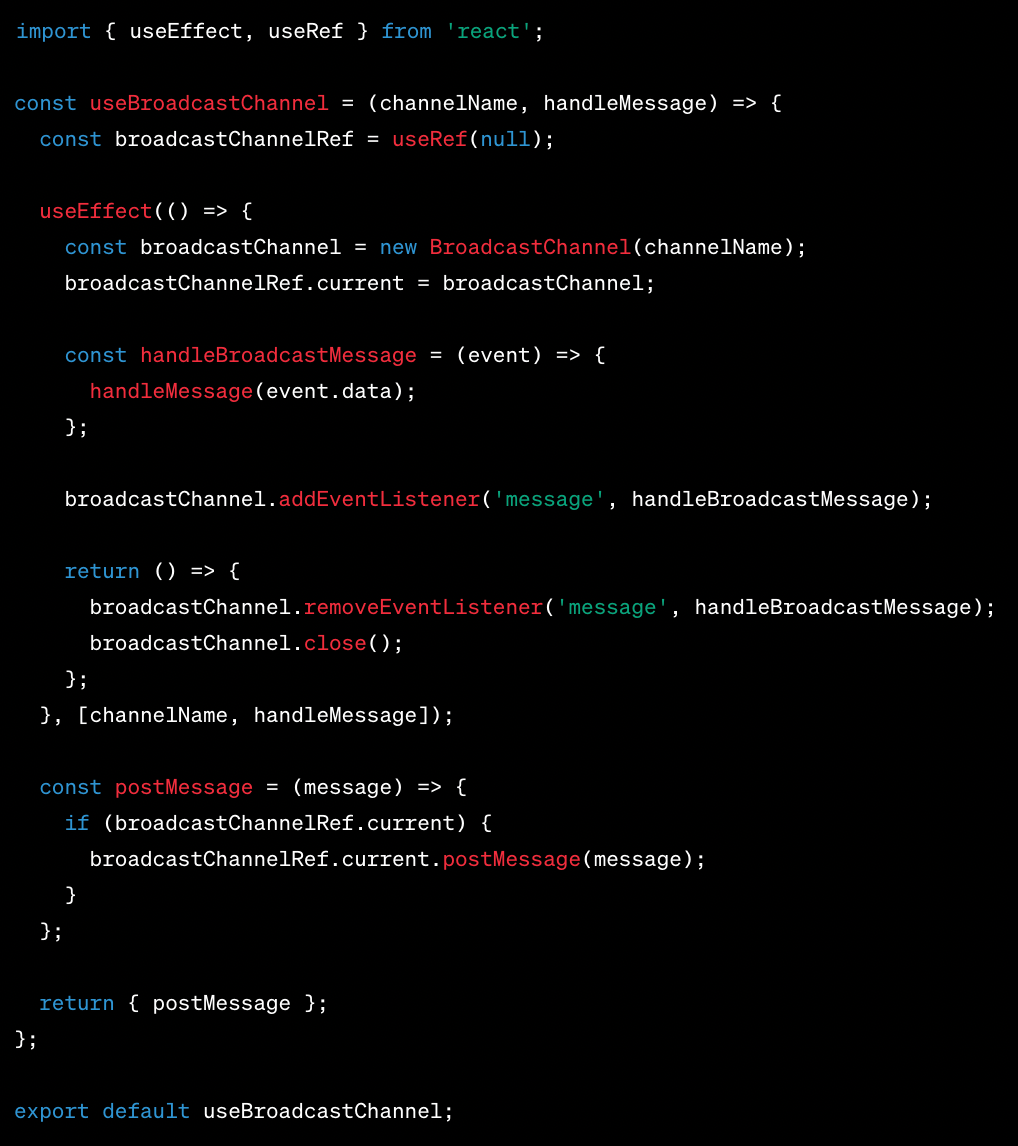
You can then use this useBroadcastChannel hook in your components like this:
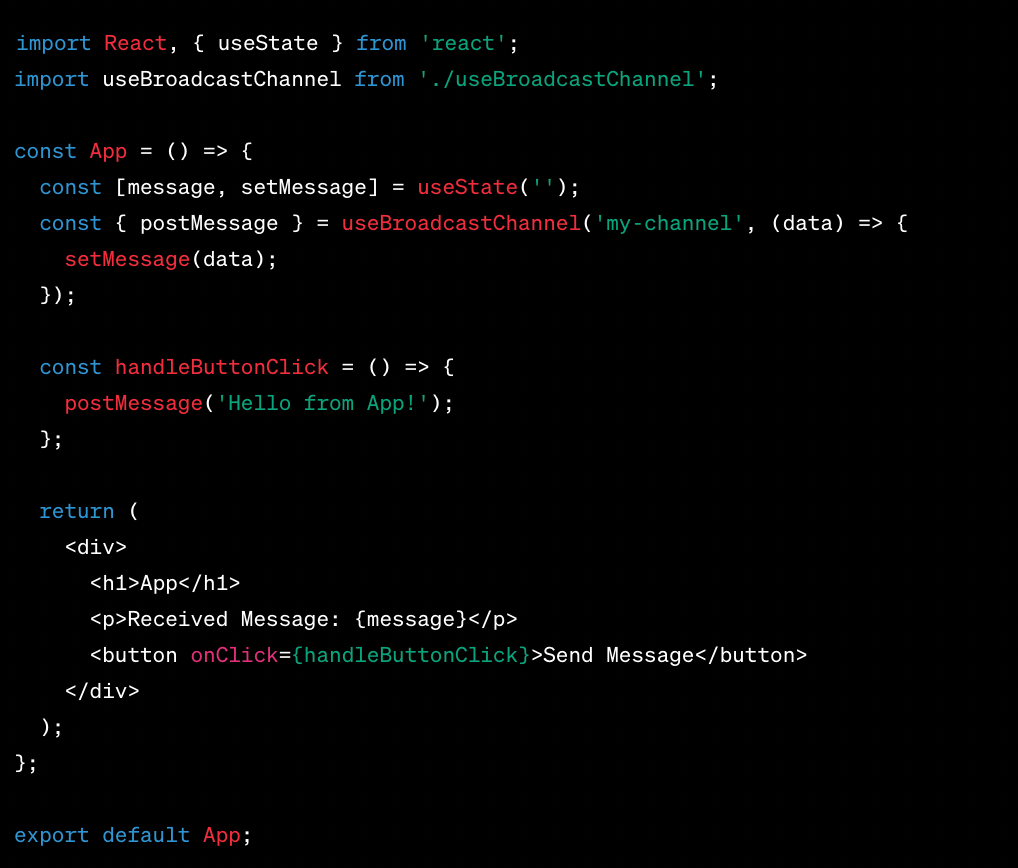
In this example, the useBroadcastChannel hook abstracts away the setup and teardown of the Broadcast Channel, allowing you to easily listen for and send messages between different tabs or windows of your application. The hook returns a postMessage function that you can use to send messages through the channel. The example usage in the App component demonstrates how you can send and receive messages using the hook.
By implementing this approach, when a user logs out in one tab, the logout event will be broadcasted to all other tabs using the Broadcast Channel API. Each tab will listen for this event and execute the logout logic in response.
Keep in mind that the Broadcast Channel API has limitations, particularly related to browser support and communication between different origins. Make sure to test thoroughly and consider fallback solutions if the API is not supported in certain browsers.
Additionally, you might want to couple this approach with proper session management, token invalidation, and backend support to ensure that even if a user logs out in one tab, their session is properly invalidated across all tabs.